Published
- 2 min read
Using Express Session for Authorization in Node.js Applications
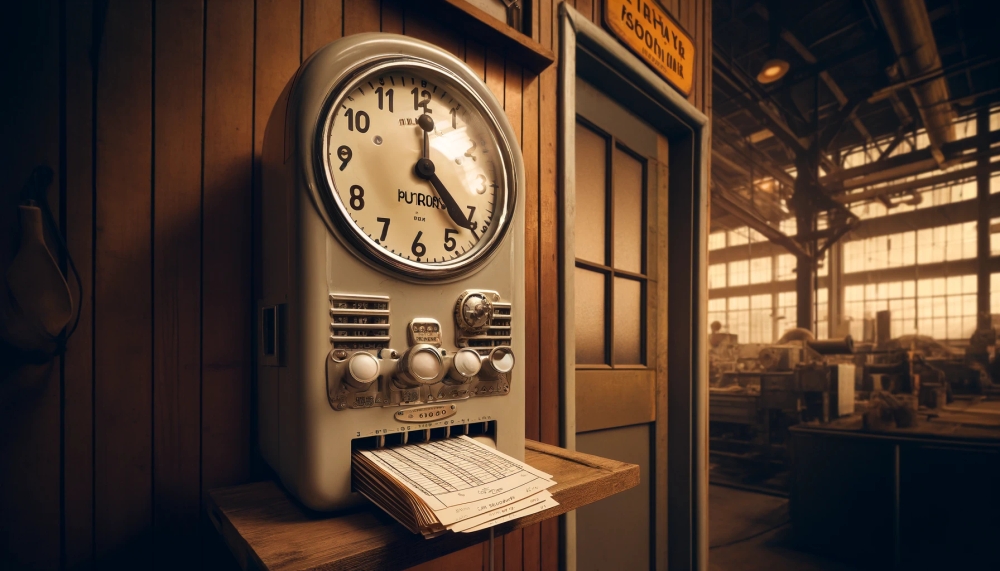
To implement Express Session in a Node.js application, you need to follow these steps:
Install the necessary dependencies:
First install Express Session:
npm install express express-session
Require the ‘express’ and ‘express-session’ modules in your Node.js application:
const express = require('express');
const session = require('express-session');
Set up the Express app and configure the session middleware.
You need to use ‘express-session’ middleware before setting up your routes:
const app = express();
app.use(session({
secret: 'your_secret_key', // Change this to your own secret
resave: false,
saveUninitialized: true
}));
secret
: This is a string used to sign the session ID cookie. You should change it to a random string.resave
: Forces the session to be saved back to the session store, even if the session was never modified during the request.saveUninitialized
: Forces a session that is “uninitialized” to be saved to the store. A session is uninitialized when it is new but not modified.
You can now access and modify the session object in your routes. Here’s an example of a route that sets a session variable and retrieves it:
app.get('/setSession', (req, res) => {
req.session.username = 'user123';
res.send('Session variable set');
});
app.get('/getSession', (req, res) => {
const username = req.session.username;
res.send('Session variable: ' + username);
});
In this example, /setSession
sets the username
session variable to 'user123'
, and /getSession
retrieves it.
Start the Express server
const port = 3000;
app.listen(port, () => {
console.log(`Server is listening on port ${port}`);
});
Make sure to handle session persistence properly, as it can be crucial for maintaining user authentication and state across requests.