Published
- 4 min read
Remediate Insecure Direct Object References (IDORs)
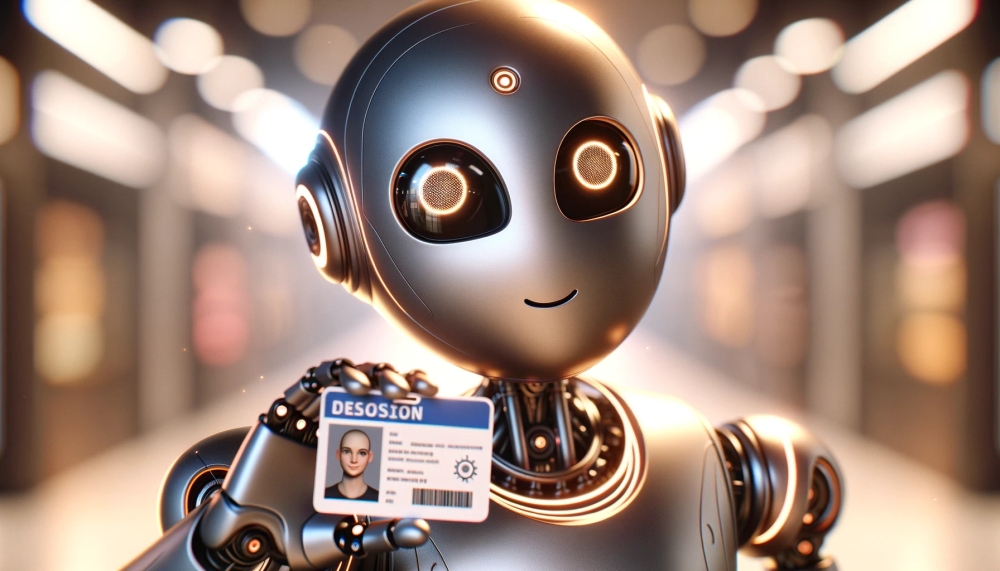
Preventing Insecure Direct Object Reference (IDOR) attacks involves implementing various security measures at different layers of your application.
ASP.NET Authorization Mechanisms for Preventing IDORs
ASP.NET provides several built-in ways to check authorization:
- Role-Based Authorization: ASP.NET supports role-based authorization, where access to resources is based on the roles assigned to users. You can use the AuthorizeAttribute to apply role-based authorization at the controller or action level in MVC or Web API applications. For example:
[Authorize(Roles = "Admin")]
public ActionResult AdminDashboard()
{
// Code for admin dashboard
}
- Identity-based Authorization: ASP.NET Identity is a membership system that provides authentication and authorization functionality. It allows you to manage users, roles, and claims, and you can use these identities to enforce authorization rules. You can check for specific roles or claims using methods provided by the User or HttpContext objects. For example:
if (User.IsInRole("Admin"))
{
// Code for admin functionality
}
- Policy-Based Authorization: ASP.NET Core introduced policy-based authorization, which allows you to define authorization policies with specific requirements and apply them to controllers or actions using the AuthorizeAttribute. Policies can be based on roles, claims, or custom requirements. For example:
services.AddAuthorization(options =>
{
options.AddPolicy("RequireAdminRole", policy =>
policy.RequireRole("Admin"));
});
Then, you can apply this policy in a controller or action:
[Authorize(Policy = "RequireAdminRole")]
public ActionResult AdminDashboard()
{
// Code for admin dashboard
}
Resource-Based Authorization:
ASP.NET Core also supports resource-based authorization, where access control is based on the properties of the resource being accessed. You can define authorization handlers that evaluate resource-specific policies and requirements. This allows for more granular control over access to resources based on their properties.
These are some of the built-in ways ASP.NET provides for checking authorization. Depending on your application’s requirements and the version of ASP.NET you’re using, you can choose the most appropriate approach to implement authorization checks effectively.
Node.js Authorization
In Node.js, there are several authorization mechanisms you can use to control access to resources in your application. Libraries like Casbin or implementing custom ABAC logic can be used to implement ABAC in Node.js applications. Custom middleware functions can be applied globally to all routes or selectively to specific routes or route patterns. Middleware can be used to verify and decode the JWT, extract user information, and perform authorization checks based on the token contents.
Express Session
Express Session is a middleware for Node.js applications built with Express.js. It facilitates session management by providing a simple API to create and manage user sessions. With Express Session, developers can easily store session data on the server-side, enabling features like user authentication, maintaining user state, and storing user-specific information across multiple requests. It utilizes cookies to uniquely identify each client and securely store session data, offering flexibility in configuration options such as session storage, expiration, and encryption. Overall, Express Session simplifies session handling in Express.js applications, making it an essential tool for building web applications with user interaction.
Passport.js:
Passport.js is a popular authentication middleware for Node.js applications. It supports various authentication strategies, including JWT, OAuth, OpenID, and more. Passport.js can be used to handle user authentication and integrate with external authentication providers (e.g., Google, Facebook, GitHub). Once authenticated, Passport.js can populate user information in the request object, which can be used for authorization checks.
Role-Based Access Control (RBAC):
- RBAC is a method for restricting access to resources based on users’ roles and permissions.
- You can implement RBAC logic in your Node.js application by defining roles and permissions and checking them during authorization checks.
- Libraries like AccessControl can help implement RBAC in Node.js applications.
Attribute-Based Access Control (ABAC):
- ABAC is an access control model that evaluates access based on attributes of the user, resource, and environment.
- ABAC policies define rules based on attributes, and access decisions are made based on these rules.
- Libraries like Casbin or implementing custom ABAC logic can be used to implement ABAC in Node.js applications.
These authorization mechanisms can be implemented using various libraries, frameworks, and middleware in Node.js, depending on your application’s requirements and preferences.