Published
- 2 min read
Using Passport.js for Authorization in Node.js Applications
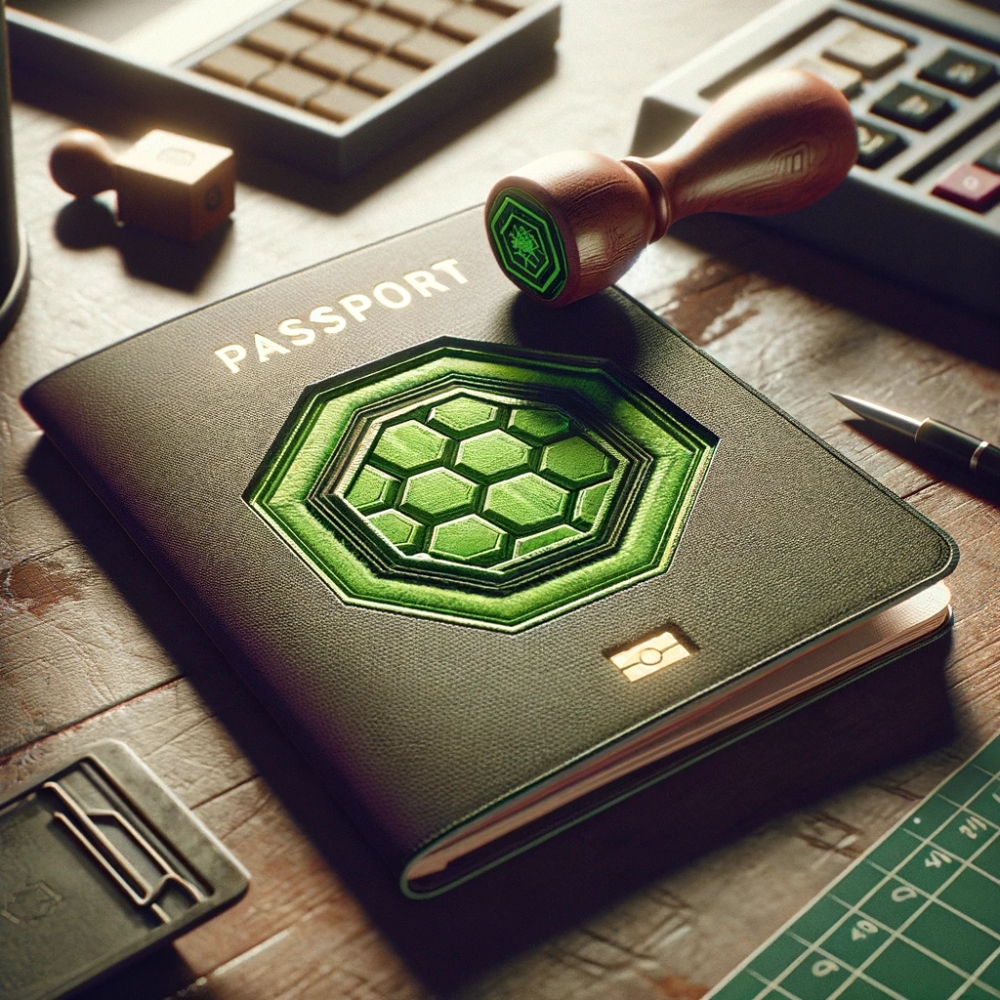
Using Passport.js for authorization involves setting up authentication strategies and middleware to authenticate users and protect routes based on their authentication status and roles. Here’s a basic example of how you can use Passport.js for authorization in a Node.js application:
Install Passport.js and relevant authentication strategies**:
First, you need to install Passport.js and any authentication strategies you want to use. For example, if you want to use local username/password authentication, you would install passport-local
:
npm install passport passport-local
Configure Passport.js
Set up Passport.js in your application, configure authentication strategies, and initialize Passport middleware.
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
// Define a local authentication strategy
passport.use(new LocalStrategy(
(username, password, done) => {
// Implement your own logic to authenticate users
// Check username and password against your database
// Call done(err, user) with the user object if authenticated, or false if not
// Example:
User.findOne({ username: username }, (err, user) => {
if (err) { return done(err); }
if (!user || !user.validPassword(password)) {
return done(null, false, { message: 'Incorrect username or password.' });
}
return done(null, user);
});
}
));
// Serialize/deserialize user functions
passport.serializeUser((user, done) => {
done(null, user.id);
});
passport.deserializeUser((id, done) => {
User.findById(id, (err, user) => {
done(err, user);
});
});
Initialize Passport Middleware
In your application’s initialization code (e.g., app.js
), initialize Passport middleware.
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const app = express();
// Set up session middleware
app.use(session({
secret: 'your_secret_key',
resave: false,
saveUninitialized: false
}));
// Initialize Passport and restore authentication state, if any, from the session
app.use(passport.initialize());
app.use(passport.session());
Protect Routes with Passport Middleware
Use Passport.js middleware to protect routes that require authentication or specific roles.
// Example route that requires authentication
app.get('/profile', isLoggedIn, (req, res) => {
res.render('profile', { user: req.user });
});
// Middleware to check if user is authenticated
function isLoggedIn(req, res, next) {
if (req.isAuthenticated()) {
return next();
}
res.redirect('/login');
}
Authenticate users
Implement login and logout routes to authenticate users using Passport.js.
app.post('/login',
passport.authenticate('local', {
successRedirect: '/profile',
failureRedirect: '/login',
failureFlash: true // Enable flash messages for failed authentication
})
);
app.get('/logout', (req, res) => {
req.logout();
res.redirect('/');
});
This is a basic example of how to use Passport.js for authorization in a Node.js application. Depending on your application’s requirements, you may need to customize the authentication strategies, middleware, and routes accordingly.